Python Tips to Make Your Code Shine! ✨

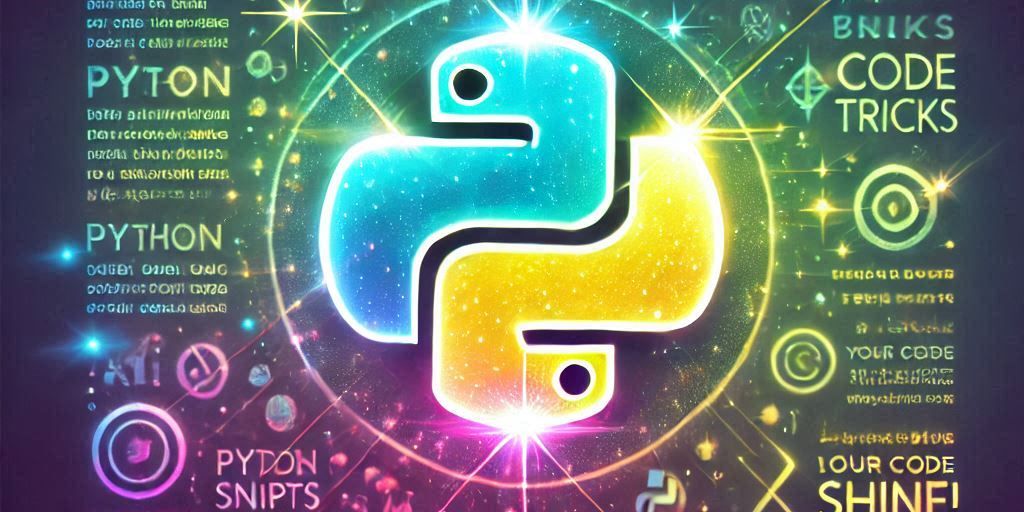

Use Descriptive Variable Names: Always use meaningful names for your variables to improve code readability.
Keep Your Code DRY: Don’t Repeat Yourself; use functions or loops to eliminate repetitive code.
Leverage Python Libraries: Explore Python's vast library ecosystem for tasks like data analysis, visualization, or web development.
Follow PEP 8 Guidelines: Stick to Python's style guide for clean and consistent code formatting.
Use List Comprehensions: Replace loops with list comprehensions for more concise and readable code.
Implement Error Handling: Use try-except blocks to handle exceptions gracefully.
Comment Smartly: Write meaningful comments to explain complex logic but avoid over-commenting obvious code.
Use F-Strings for Strings: Simplify string formatting using f-strings for cleaner syntax.
Practice Modular Coding: Break your code into smaller functions or modules for better organization.
Use Python's Built-in Functions: Maximize efficiency with built-in functions like sum()
, len()
, and sorted()
.
Learn Pythonic Ways: Embrace Python-specific idioms like zip()
or enumerate()
for cleaner code.
Optimize with Generators: Use generators for large datasets to save memory.
Use Type Hints: Add type hints to functions for better readability and error prevention.
Test Your Code: Write unit tests to ensure your code behaves as expected.
Avoid Global Variables: Keep variables local to improve code maintainability and prevent conflicts.
Master Dictionary Comprehensions: Simplify dictionary creation with concise comprehensions.
Use Virtual Environments: Isolate your projects to avoid dependency conflicts.
Keep Your Code Clean: Use tools like black
or flake8
to format and lint your code.
Learn Context Managers: Use with
statements to handle resources like files or database connections.
Understand Mutable vs Immutable: Know when to use mutable (lists) or immutable (tuples) types.
Profile Your Code: Identify bottlenecks using tools like cProfile
or timeit
.
Use Decorators: Simplify repetitive function patterns with decorators.
Practice Code Reviews: Regularly review your code or get it reviewed for improvements.
Stay Updated: Keep up with Python updates to utilize new features and improvements.
Write Reusable Code: Design functions and classes that can be reused across multiple projects.